SpacetimeDB Documentation
Installation
You can run SpacetimeDB as a standalone database server via the spacetime CLI tool.
You can find the instructions to install the CLI tool for your platform here.
To get started running your own standalone instance of SpacetimeDB check out our Getting Started Guide.
What is SpacetimeDB?
You can think of SpacetimeDB as a database that is also a server.
It is a relational database system that lets you upload your application logic directly into the database by way of very fancy stored procedures called "modules".
Instead of deploying a web or game server that sits in between your clients and your database, your clients connect directly to the database and execute your application logic inside the database itself. You can write all of your permission and authorization logic right inside your module just as you would in a normal server.
This means that you can write your entire application in a single language, Rust, and deploy it as a single binary. No more microservices, no more containers, no more Kubernetes, no more Docker, no more VMs, no more DevOps, no more infrastructure, no more ops, no more servers.
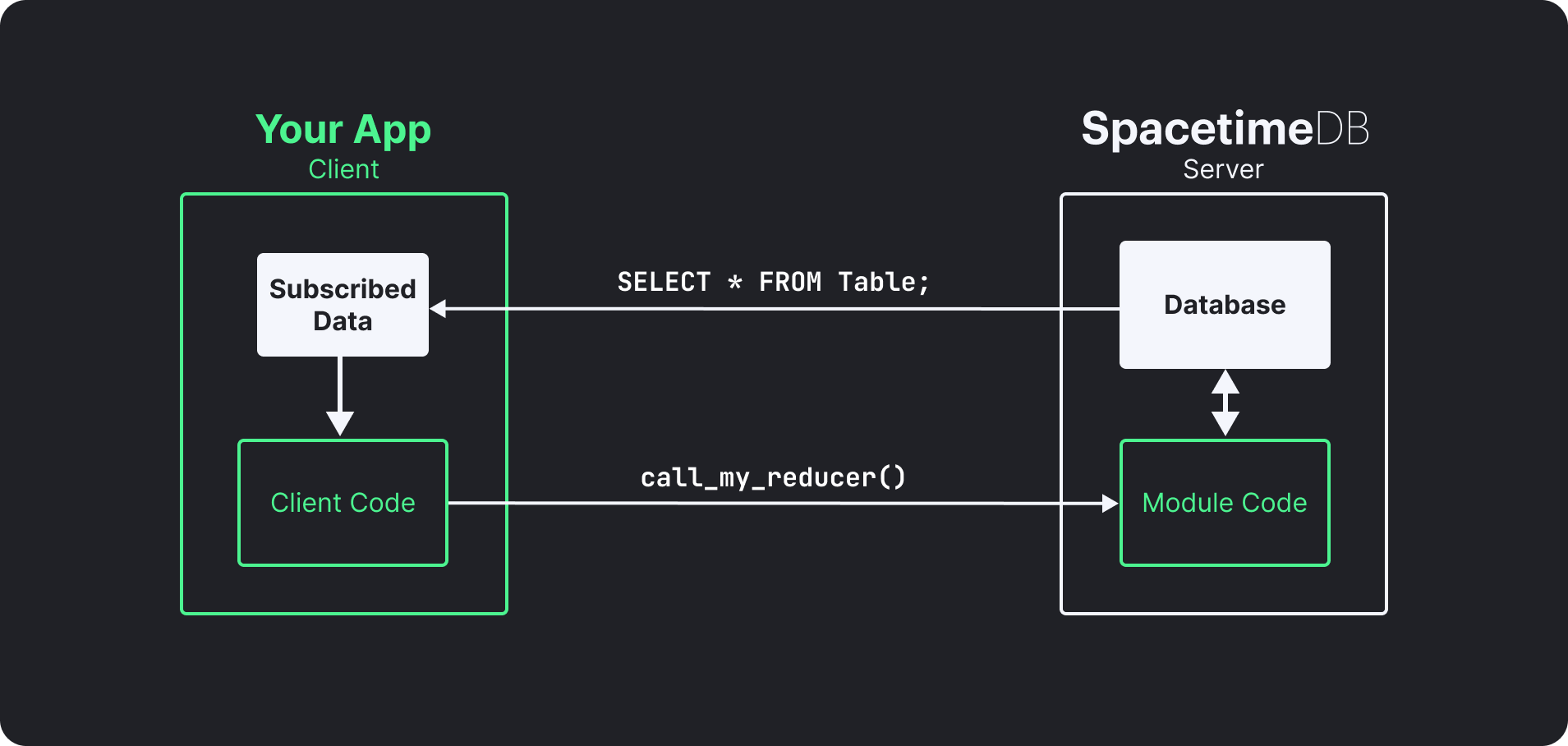
It's actually similar to the idea of smart contracts, except that SpacetimeDB is a database, has nothing to do with blockchain, and it's a lot faster than any smart contract system.
So fast, in fact, that the entire backend our MMORPG BitCraft Online is just a SpacetimeDB module. We don't have any other servers or services running, which means that everything in the game, all of the chat messages, items, resources, terrain, and even the locations of the players are stored and processed by the database before being synchronized out to all of the clients in real-time.
SpacetimeDB is optimized for maximum speed and minimum latency rather than batch processing or OLAP workloads. It is designed to be used for real-time applications like games, chat, and collaboration tools.
This speed and latency is achieved by holding all of application state in memory, while persisting the data in a write-ahead-log (WAL) which is used to recover application state.
State Synchronization
SpacetimeDB syncs client and server state for you so that you can just write your application as though you're accessing the database locally. No more messing with sockets for a week before actually writing your game.
Identities
A SpacetimeDB Identity is a unique identifier that is used to authenticate and authorize access to the database. Importantly, while it represents who someone is, does NOT represent what they can do. Your application's logic will determine what a given identity is able to do by allowing or disallowing a transaction based on the caller's Identity along with any module-defined data and logic.
SpacetimeDB associates each user with a 256-bit (32-byte) integer Identity. These identities are usually formatted as 64-digit hexadecimal strings. Identities are public information, and applications can use them to identify users. Identities are a global resource, so a user can use the same identity with multiple applications, so long as they're hosted by the same SpacetimeDB instance.
Each identity has a corresponding authentication token. The authentication token is private, and should never be shared with anyone. Specifically, authentication tokens are JSON Web Tokens signed by a secret unique to the SpacetimeDB instance.
Additionally, each database has an owner Identity. Many database maintenance operations, like publishing a new version or evaluating arbitrary SQL queries, are restricted to only authenticated connections by the owner.
SpacetimeDB provides tools in the CLI and the client SDKs for managing credentials.
Addresses
A SpacetimeDB Address is an opaque identifier for a database or a client connection. An Address is a 128-bit integer, usually formatted as a 32-character (16-byte) hexadecimal string.
Each SpacetimeDB database has an Address, generated by the SpacetimeDB host, which can be used to connect to the database or to request information about it. Databases may also have human-readable names, which are mapped to addresses internally.
Each client connection has an Address. These addresses are opaque, and do not correspond to any metadata about the client. They are notably not IP addresses or device identifiers. A client connection can be uniquely identified by its (Identity, Address) pair, but client addresses may not be globally unique; it is possible for multiple connections with the same Address but different identities to co-exist. SpacetimeDB modules should treat Identity as differentiating users, and Address as differentiating connections by the same user.
Language Support
Server-side Libraries
Currently, Rust is the best-supported language for writing SpacetimeDB modules. Support for lots of other languages is in the works!
- Rust - (Quickstart)
- C# - (Quickstart)
- Python (Coming soon)
- Typescript (Coming soon)
- C++ (Planned)
- Lua (Planned)
Client-side SDKs
- Rust - (Quickstart)
- C# - (Quickstart)
- TypeScript - (Quickstart)
- Python (Planned)
- C++ (Planned)
- Lua (Planned)
Unity
SpacetimeDB was designed first and foremost as the backend for multiplayer Unity games. To learn more about using SpacetimeDB with Unity, jump on over to the SpacetimeDB Unity Tutorial.
FAQ
What is SpacetimeDB? It's a whole cloud platform within a database that's fast enough to run real-time games.
How do I use SpacetimeDB? Install the spacetime command line tool, choose your favorite language, import the SpacetimeDB library, write your application, compile it to WebAssembly, and upload it to the SpacetimeDB cloud platform. Once it's uploaded you can call functions directly on your application and subscribe to changes in application state.
How do I get/install SpacetimeDB? Just install our command line tool and then upload your application to the cloud.
How do I create a new database with SpacetimeDB? Follow our Quick Start guide!
TL;DR in an empty directory:
spacetime init --lang=rust
spacetime publish
- How do I create a Unity game with SpacetimeDB? Follow our Unity Project guide!
TL;DR in an empty directory:
spacetime init --lang=rust
spacetime publish
spacetime generate --out-dir <path-to-unity-project> --lang=csharp